HTTP API Documentation
Through the HTTP API documentation you will learn how you can control your video scoreboard remotely, either through a Stream Deck type device, a curl command call through the command line, or through software that you develop yourself.
What is an API and an HTTP API?
An API (Application Programming Interface) is an element that provides a way for other systems to interact with it. There are many types of API, which is why I’ve referred to it as an “element”, and it can provide many types of interactions: you can send data to it, collect data from that system, or control it remotely.
In our case, we have implemented an HTTP API , which means that we have a kind of web server, which is waiting to receive your requests as if you were requesting a website, and do things based on the address you typed.
So the important part here is that it’s a web server, so you can send HTTP API requests using any program that can request web pages (your favorite browser, the application curl on Linux and OSX, etc.) and, more importantly, you can do it from any computer, tablet, or cell phone that is on the same network allowing you to have multiple LDSscoreboards (or LDSperimetral) controlled from a centralized point, your control center.
To call this HTTP API, you must consult a url (URL is the name given to the address made up of the protocol, computer, port and request) as if it were a “web page”. But where is that web page? I’m making a mess!. The easiest way is to open the request you want with your preferred browser. The address to put will have the following form: https://COMPUTERS_IP:CONFIGURED_PORT/REQUEST
Therefore, and in summary:
- the IP of the computer depends on the network configuration of the equipment. We recommend that you make it fixed to prevent it from changing and the requests from going to another computer without realizing it.
- The port is indicated in the terminal configuration, marked with a red arrow.
- The request will depend on the order you want to give to LDSscoreboard (we will see it shortly).
Through this HTTP API documentation you will learn how to control the video scoreboard remotely in a simple way.
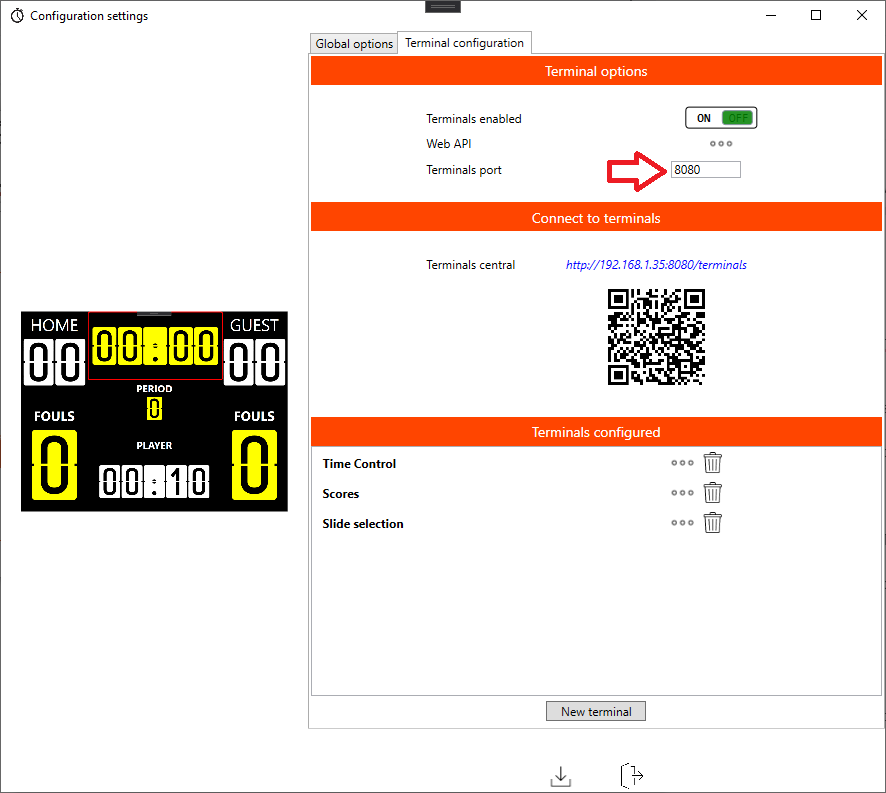
In the case shown, the IP of our computer is 192.168.1.35 and the configured port is 8080.
API Description
The goal is to have an API for all LDSscoreboard control commands except content editing. In this way you can centrally control all the screens you want, publish promotional content or bookmark elements.
slide control
Command | Parameters |
---|---|
/pcontrol/show_slide/<SLIDE_NAME> | Causes the indicated slide to be displayed on the screen.
name_slide = Name of the slide that you want to show |
Counter control
Command | Parameters |
---|---|
/pcontrol/increment_counter/COUNTER_NAME | Increments the counter passed as a parameter
counter_name = Name of the counter on which to operate |
/pcontrol/decrement_counter/COUNTER_NAME | Decrements the counter passed as a parameter
counter_name = Name of the counter on which to operate |
/pcontrol/set_counter/COUNTER_NAME/VALUE | Assigns a value to a given counter.
counter_name = Name of the counter on which to operate |
/pcontrol/get_counter_value/COUNTER_NAME | Retrieves the value of a counter.
counter_name = Name of the counter on which to operate |
player’s roster
Command | Parameters |
---|---|
/roster/get_local_team_name | Check the local computer name |
/roster/get_guest_team_name | Check the name of the away team |
/roster/get_team_players_count/[local|guest] | Returns the number of players in the home or away team |
/roster/get_player_information/PLAYER_INDEX[local|guest] | Returns the information of the indicated player (name, number, etc..) |
/roster/increment_player_score/[local|guest] /PLAYER_INDEX | Increases the score associated with the player with the indicated index |
/roster/decrement_player_score/[local|guest] /PLAYER_INDEX | Decreases the score associated with the player with the indicated index |
/roster/get_player_score/[local|guest] /PLAYER_INDEX | Retrieves the score associated with the player with the indicated index |
/roster/increment_player_fauls/[local|guest] /PLAYER_INDEX | Increases the fouls associated with the player with the indicated index |
/roster/decrement_player_fauls/[local|guest] /PLAYER_INDEX | Decreases the fouls associated with the player with the indicated index |
/roster/get_player_faults/[local|guest] /PLAYER_INDEX | Recovers the faults associated with the player with the indicated index |
/roster/increment_player_cautions/[local|guest] /PLAYER_INDEX | Increases the warnings associated with the player with the indicated index |
/roster/decrement_player_cautions/[local|guest] /PLAYER_INDEX | Decreases the warnings associated with the player with the indicated index |
/roster/get_player_cautions/[local|guest] /PLAYER_INDEX | Retrieves the warnings associated with the player with the indicated index |
timer control
Command | Parameters |
---|---|
/pcontrol/start_timer/TIMER_NAME | Starts the timer in the direction in which it has been defined.
name_timer = Name of the timer (or chronometer) on which to operate |
/pcontrol/stop_timer/TIMER_NAME | Stops the timer. Its value is not altered.
name_timer = Name of the timer (or chronometer) on which to operate |
/pcontrol/reset_timer/TIMER_NAME | Resets the timer to the initial value defined in its configuration.
name_timer = Name of the timer (or chronometer) on which to operate |
/pcontrol/get_timer_time/TIMER_NAME | Recovers the timer’s value of a timer.
name_timer = Name of the timer (or chronometer) on which to operate |
Dynamic labels and automatic counters
Command | Parameters |
---|---|
/gc/get_dynamic_label/VAR | Retrieves the value assigned to the dynamic variable
VAR = Identifier of the variable to be queried |
/gc/set_dynamic_label/VAR/ARG1 | Changes the value assigned to the dynamic variable
VAR = Identifier of the variable to be assigned |
/gc/get_automatic_variable/VAR | Gets the value of an automatic variable. These variables are calculated when using the player roster.
VAR = Variable name Existing variables: |
/gc/reset_automatic_variable/VAR | Resets the counter to zero for automatic variables that can be reset.
VAR: Name of the resettable variable (local_team_fauls_resetable or guest_team_fauls_resetable) |
LDSscoreboard HTTP API usage example
Let’s see an example to get a clear understanding of the operation described in this documentation on the HTTP API. Suppose we have defined a marker that contains a slide called “slide1”, to request the system to open slide1, we should know the IP of the computer and the port, since we already know the name.
If we take the image at the top of the page, we will see that we have the ip 192.168.1.35 and port 8080, so we should make an HTTP request (open the page) to the address http://192.168.1.35:8080 /pcontrol/show_slide/slide1
To do so, we can open a browser and put the address in the address bar:
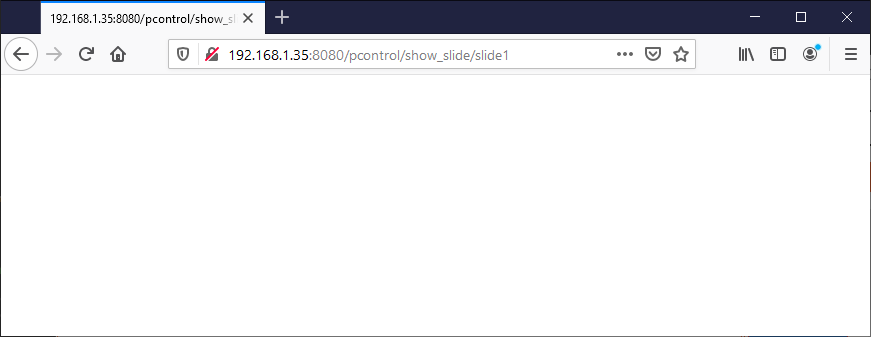
Next, if we have LDSscoreboard running we should see the slide called slide1 become active.
IMPORTANT
If the name of the slide contains a space, being a special character, we must replace it with %20, that is, “my slide” will be translated as “my%20slide”.
Tools for making HTTP API calls
Of course, you are not restricted to having to make HTTP calls from a browser, we have done it this way because it is a tool that you surely have at hand and you know, but there are many other ways to do it.
curl
curl is an open source HTTP request tool. It is available on Linux, OSX, and also on the latest versions of Windows 10. Its use is very simple as seen in the following image:

JavaScript
You can make a program in Javascript, using jquery, where an HTTP call is made, for example with this code:
timer_identifier=”my timer”;
$.getJSON(`/pcontrol/start_
.done(function (data) {
if (data.status != 0) {
console.error(`Error starting timer: ${JSON.stringify(data)} `);
}
})
.fail(function (err) {
if (err.status != 200)
console.error(`Error starting timer [${JSON.stringify(err)}]`);
});
and any programming language will allow you to make these types of calls.